The Kubernetes orchestrator is a marvel of software engineering; it allows us to deploy, manage and scale our applications without many headaches. However, it's a tool in the end, and how you use it dictates how well you sleep at night. If you don't treat it well, it will keep you up at night, but if you treat it well and maintain it correctly, you can sleep well. Where in this analogy comes the authorization part? Well, suppose you're not managing access to your clusters. In that case, anybody with access can make honest mistakes or perform malicious actions, which can cause all the security problems we're all aware of.
In this article, we will discuss the two primary authorization models in AKS—native Kubernetes RBAC and Azure RBAC integration—exploring their inner workings and differences and providing practical implementation examples you can apply to your environment today.
Understanding Authorization Fundamentals in Kubernetes
Before we dive into the specifics of each authorization model, let's define what authorization means in the context of Kubernetes. Authorization determines whether an authenticated user, group, or service account has permission to perform a specific action on a particular resource. This is different from authentication, which only verifies identity.
In Kubernetes, authorization decisions are based on four key elements:
- Who is making the request (a user, group, or service account)
- What action are they attempting to perform (get, list, create, update, delete, etc.)?
- Which resource are they trying to act upon (pods, deployments, services, etc.)?
- Where the resource is located (which namespace)
For AKS clusters, you have two primary options for implementing authorization:
- Kubernetes RBAC: The native Role-Based Access Control system that comes with Kubernetes
- Azure RBAC: Azure's built-in Role-Based Access Control system extended to include Kubernetes resources
I remember when Azure RBAC was first announced and was available as a preview. I hated it at first, but once I understood it better, I was sold; you have RBAC and set up PIM for escalation, perfect for security. But that doesn't automatically make it the right choice for everyone. Let's explore both models in detail so you can make an informed decision for your environment.
Kubernetes RBAC: The Native Approach
Kubernetes Role-Based Access Control (RBAC) has been part of Kubernetes since version 1.6 and became stable in version 1.8. It's the native method of regulating access to Kubernetes resources based on the roles of individual users within your organization.
Core Components of Kubernetes RBAC
RBAC in Kubernetes is built around four primary API objects:
- Roles: Define permissions within a specific namespace
- ClusterRoles: Define permissions across the entire cluster (not namespace-specific)
- RoleBindings: Bind Roles to users, groups, or service accounts within a specific namespace
- ClusterRoleBindings: Bind ClusterRoles to users, groups, or service accounts across the entire cluster
Roles and ClusterRoles
A Role contains a set of rules that represent a set of permissions. These permissions are purely additive (there are no "deny" rules). Here's a simple example of a Role that allows reading pods in a namespace:
apiVersion: rbac.authorization.k8s.io/v1
kind: Role
metadata:
namespace: default
name: pod-reader
rules:
- apiGroups: [""]
resources: ["pods"]
verbs: ["get", "watch", "list"]
ClusterRoles work similarly but are not namespace scoped. They're useful for:
- Cluster-wide resources (like nodes)
- Non-resource endpoints (like /healthz)
- Resources in all namespaces
Here's an example of a ClusterRole that allows reading secrets across all namespaces:
apiVersion: rbac.authorization.k8s.io/v1
kind: ClusterRole
metadata:
name: secret-reader
rules:
- apiGroups: [""]
resources: ["secrets"]
verbs: ["get", "watch", "list"]
One aspect of ClusterRoles that often confuse engineers is that although they are cluster-wide in definition when bound with a RoleBinding (not a ClusterRoleBinding), the permissions are limited to the namespace of that RoleBinding.
Understanding Rules in Detail
The rules in Roles and ClusterRoles are composed of three essential elements:
- apiGroups: Specifies the API groups containing the resources you want to grant access to. Core resources like pods and services belong to the "" (empty) API group, while extensions like deployments belong to the "apps" API group.
- resources: Defines the resource types you're granting access to, such as pods, services, deployments, etc.
- verbs: Specifies the actions that can be performed on the resources. Common verbs include get, list, create, update, patch, delete, and deletecollection.
For more specific control, you can also use resourceNames to restrict actions to particular instances of a resource:
apiVersion: rbac.authorization.k8s.io/v1
kind: Role
metadata:
namespace: default
name: configmap-updater
rules:
- apiGroups: [""]
resources: ["configmaps"]
resourceNames: ["my-configmap"]
verbs: ["update", "get"]
This Role only allows getting and updating a ConfigMap named "my-configmap" in the default namespace.
RoleBindings and ClusterRoleBindings
Once you've defined your Roles and ClusterRoles, you need to bind them to users, groups, or service accounts using RoleBindings and ClusterRoleBindings.
A RoleBinding grants the permissions defined in a Role or ClusterRole to a user, group, or service account within a specific namespace:
apiVersion: rbac.authorization.k8s.io/v1
kind: RoleBinding
metadata:
name: read-pods
namespace: default
subjects:
- kind: User
name: florin
apiGroup: rbac.authorization.k8s.io
roleRef:
kind: Role
name: pod-reader
apiGroup: rbac.authorization.k8s.io
This RoleBinding grants the permissions defined in the "pod-reader" Role to a user named "florin" within the default namespace.
A ClusterRoleBinding grants permissions defined in a ClusterRole across the entire cluster:
apiVersion: rbac.authorization.k8s.io/v1
kind: ClusterRoleBinding
metadata:
name: read-secrets-global
subjects:
- kind: Group
name: managers
apiGroup: rbac.authorization.k8s.io
roleRef:
kind: ClusterRole
name: secret-reader
apiGroup: rbac.authorization.k8s.io
This ClusterRoleBinding grants the permissions defined in the "secret-reader" ClusterRole to all users in the "managers" group across all namespaces.
Team-Based Access in AKS
Let's look at a practical example of implementing Kubernetes RBAC in an AKS cluster for team-based access control. I've implemented this scenario many times for enterprise clients.
First, we'll create the necessary namespaces for each team:
kubectl create namespace team-a
kubectl create namespace team-b
Next, we'll create a ClusterRole for read-only access:
apiVersion: rbac.authorization.k8s.io/v1
kind: ClusterRole
metadata:
name: namespace-reader
rules:
- apiGroups: ["", "apps", "batch", "extensions"]
resources: ["*"]
verbs: ["get", "list", "watch"]
And another ClusterRole for read-write access:
apiVersion: rbac.authorization.k8s.io/v1
kind: ClusterRole
metadata:
name: namespace-admin
rules:
- apiGroups: ["", "apps", "batch", "extensions"]
resources: ["*"]
verbs: ["*"]
Now, we need to create RoleBindings to grant these permissions to our teams. First, let's grant Team A admin access to their namespace:
apiVersion: rbac.authorization.k8s.io/v1
kind: RoleBinding
metadata:
name: team-a-admin
namespace: team-a
subjects:
- kind: Group
name: "00000000-0000-0000-0000-000000000001" # Microsoft Entra ID Group Object ID for Team A
apiGroup: rbac.authorization.k8s.io
roleRef:
kind: ClusterRole
name: namespace-admin
apiGroup: rbac.authorization.k8s.io
And let's grant Team B admin access to their namespace:
apiVersion: rbac.authorization.k8s.io/v1
kind: RoleBinding
metadata:
name: team-b-admin
namespace: team-b
subjects:
- kind: Group
name: "00000000-0000-0000-0000-000000000002" # Microsoft Entra ID Group Object ID for Team B
apiGroup: rbac.authorization.k8s.io
roleRef:
kind: ClusterRole
name: namespace-admin
apiGroup: rbac.authorization.k8s.io
Additionally, we might want to grant Team A read-only access to Team B's namespace for collaboration:
apiVersion: rbac.authorization.k8s.io/v1
kind: RoleBinding
metadata:
name: team-a-reader
namespace: team-b
subjects:
- kind: Group
name: "00000000-0000-0000-0000-000000000001" # Microsoft Entra ID Group Object ID for Team A
apiGroup: rbac.authorization.k8s.io
roleRef:
kind: ClusterRole
name: namespace-reader
apiGroup: rbac.authorization.k8s.io
With these RoleBindings in place, Team A has full access to the team-a namespace and read-only access to the team-b namespace, while Team B has full access to only the team-b namespace.
Integrating with Microsoft Entra ID
In the examples above, we use Microsoft Entra ID (formerly Azure AD) groups for authorization. This integration allows you to leverage your identity management system for Kubernetes access control.
When you create an AKS cluster with Microsoft Entra ID integration, AKS uses OpenID Connect as the authentication method. This allows Kubernetes to validate tokens issued by Microsoft Entra ID.
To configure this integration, you first need to enable Microsoft Entra ID integration when creating your AKS cluster:
az aks create \
--resource-group myResourceGroup \
--name myAKSCluster \
--enable-aad \
--aad-admin-group-object-ids <admin-group-object-id> \
--generate-ssh-keys
This command creates an AKS cluster with Microsoft Entra ID integration enabled and grants the specified Microsoft Entra ID group administrative access to the cluster.
Once your cluster is created, you can create RoleBindings and ClusterRoleBindings referencing Microsoft Entra ID users and groups by their Object IDs, as shown in the above examples.
Azure RBAC for Kubernetes: The Integrated Approach
Azure RBAC for Kubernetes is a feature that extends Azure's built-in Role-Based Access Control system to include Kubernetes resources in AKS clusters. It was introduced to provide a more seamless and integrated authorization experience for organizations that are heavily invested in the Azure ecosystem.
I remember when Azure RBAC for Kubernetes was first announced. It was a significant step forward in simplifying authorization management for AKS clusters. Instead of maintaining separate RBAC systems for Azure and Kubernetes resources, you could now manage both through a unified interface.
How Azure RBAC for Kubernetes Works
Azure RBAC for Kubernetes works by mapping Azure role assignments to Kubernetes permissions. When a user or service principal attempts to access a Kubernetes resource, the following process occurs:
- The user or service principal authenticates with Microsoft Entra ID and receives a token.
- The token is presented to the Kubernetes API server.
- The AKS cluster, which is configured to use Azure RBAC, validates the token and checks Azure for role assignments associated with the user or service principal.
- The user or service principal is granted or denied access to the requested Kubernetes resource based on the Azure role assignments.
This integration allows you to manage Kubernetes authorization using the same tools and interfaces for other Azure resources, such as the Azure portal, Azure CLI, Azure PowerShell, or Azure Resource Manager templates.
Built-in Roles for AKS
Azure provides several built-in roles specific to AKS:
- Azure Kubernetes Service RBAC Cluster Admin: This role, similar to the Kubernetes cluster-admin role, lets you manage all resources in all namespaces.
- Azure Kubernetes Service RBAC Admin: You can manage all resources within a namespace.
- Azure Kubernetes Service RBAC Writer: Provides read-write access to most resources in a namespace.
- Azure Kubernetes Service RBAC Reader: Grants read-only access to most resources in a namespace.
- Azure Kubernetes Service Cluster User Role: This role allows access to the Kubernetes API server (required to use kubectl).
These roles we're created to cover common scenarios, but you can also create custom roles if you need more specific permissions.
Setting Up Azure RBAC for Kubernetes
To use Azure RBAC for Kubernetes, you need to enable it when creating your AKS cluster or update an existing cluster:
# Creating a new cluster with Azure RBAC enabled
az aks create \
--resource-group myResourceGroup \
--name myAKSCluster \
--enable-aad \
--enable-azure-rbac
# Updating an existing cluster to enable Azure RBAC
az aks update \
--resource-group myResourceGroup \
--name myAKSCluster \
--enable-azure-rbac
Note that enabling Azure RBAC requires Microsoft Entra ID integration (--enable-aad flag).
Once Azure RBAC is enabled, you can start assigning roles to users and groups using Azure's standard role assignment methods:
# Assigning the AKS RBAC Writer role to a user for a specific namespace
az role assignment create \
--assignee "[email protected]" \
--role "Azure Kubernetes Service RBAC Writer" \
--scope "/subscriptions/<subscription-id>/resourceGroups/<resource-group>/providers/Microsoft.ContainerService/managedClusters/<cluster-name>/namespaces/<namespace>"
# Assigning the AKS RBAC Cluster Admin role to a group for the entire cluster
az role assignment create \
--assignee-object-id "<group-object-id>" \
--assignee-principal-type Group \
--role "Azure Kubernetes Service RBAC Cluster Admin" \
--scope "/subscriptions/<subscription-id>/resourceGroups/<resource-group>/providers/Microsoft.ContainerService/managedClusters/<cluster-name>"
Team-Based Access with Azure RBAC
Let's look at a practical example of using Azure RBAC for Kubernetes to implement team-based access control, similar to the Kubernetes RBAC example above.
First, you need to ensure your AKS cluster has Azure RBAC enabled as shown above. Next, you need to create Microsoft Entra ID groups for each team (if they don't already exist):
# Create Microsoft Entra ID groups for each team
az ad group create --display-name "Team A" --mail-nickname "team-a"
az ad group create --display-name "Team B" --mail-nickname "team-b"
Then, you need to create the necessary namespaces in your AKS cluster:
kubectl create namespace team-a
kubectl create namespace team-b
Now, you can assign the appropriate roles to each team:
# Get the Object IDs of the groups
TEAM_A_ID=$(az ad group show --group "Team A" --query id -o tsv)
TEAM_B_ID=$(az ad group show --group "Team B" --query id -o tsv)
# Get the cluster resource ID
CLUSTER_ID=$(az aks show --resource-group myResourceGroup --name myAKSCluster --query id -o tsv)
# Assign admin role to Team A for their namespace
az role assignment create \
--assignee-object-id "$TEAM_A_ID" \
--assignee-principal-type Group \
--role "Azure Kubernetes Service RBAC Admin" \
--scope "$CLUSTER_ID/namespaces/team-a"
# Assign admin role to Team B for their namespace
az role assignment create \
--assignee-object-id "$TEAM_B_ID" \
--assignee-principal-type Group \
--role "Azure Kubernetes Service RBAC Admin" \
--scope "$CLUSTER_ID/namespaces/team-b"
# Assign reader role to Team A for Team B's namespace
az role assignment create \
--assignee-object-id "$TEAM_A_ID" \
--assignee-principal-type Group \
--role "Azure Kubernetes Service RBAC Reader" \
--scope "$CLUSTER_ID/namespaces/team-b"
With these role assignments in place, Team A has full access to the team-a namespace and read-only access to the team-b namespace, while Team B has full access to only the team-b namespace, just like in our Kubernetes RBAC example.
Custom Roles for Fine-Grained Control
If the built-in roles don't provide the level of granularity you need, you can create custom roles with specific permissions. Here's an example of a custom role that allows read access to pods, services, and deployments in a namespace:
{
"Name": "AKS Pod, Service, and Deployment Reader",
"Description": "Can read pods, services, and deployments in a namespace",
"Actions": [],
"NotActions": [],
"DataActions": [
"Microsoft.ContainerService/managedClusters/pods/read",
"Microsoft.ContainerService/managedClusters/services/read",
"Microsoft.ContainerService/managedClusters/apps/deployments/read"
],
"NotDataActions": [],
"AssignableScopes": [
"/subscriptions/<subscription-id>"
]
}
You can create this custom role using the Azure CLI:
az role definition create --role-definition @custom-role.json
Then, you can assign this custom role just like any other role:
az role assignment create \
--assignee "[email protected]" \
--role "AKS Pod, Service, and Deployment Reader" \
--scope "$CLUSTER_ID/namespaces/team-a"
Comparing Kubernetes RBAC and Azure RBAC
After exploring both Kubernetes RBAC and Azure RBAC in depth, let's compare these two authorization models to help you decide which one to use for your AKS clusters.
Feature Comparison
Feature | Kubernetes RBAC | Azure RBAC |
---|---|---|
Native to Kubernetes | Yes | No |
Integration with Microsoft Entra ID | Possible but requires manual mapping | Seamless |
Management Interface | kubectl, YAML files | Azure Portal, Azure CLI, Azure PowerShell, ARM templates |
Granularity | Very fine-grained | Fine-grained but limited to pre-defined actions |
Deny Rules | No | No (same as Kubernetes RBAC) |
Scope Levels | Namespace or Cluster | Subscription, Resource Group, Cluster, or Namespace |
Auditing Capabilities | Limited | Extensive through Azure Activity Logs |
Policy Enforcement | Requires additional tools | Integrated with Azure Policy |
Custom Roles | Yes, through custom Roles and ClusterRoles | Yes, through custom Azure role definitions |
When to Use Kubernetes RBAC
I recommend using Kubernetes RBAC when:
- You need maximum flexibility: Kubernetes RBAC offers the most fine-grained control over permissions.
- You're using a multi-cloud strategy: Kubernetes RBAC works the same way across all Kubernetes distributions, making it easier to maintain consistent authorization policies across multiple environments.
- You have existing Kubernetes RBAC policies: If you're migrating from another Kubernetes platform to AKS, you can bring your existing RBAC policies.
- You have specialized requirements: Some Kubernetes features and third-party operators expect or require specific Kubernetes RBAC configurations.
When to Use Azure RBAC
I recommend using Azure RBAC when:
- You need hierarchical scoping: Azure RBAC allows you to define roles at various levels (subscription, resource group, cluster, namespace), which can simplify management in complex environments.
- Audit and compliance are critical: Azure's extensive auditing capabilities make tracking and reporting on authorization activities easier.
- You want to leverage Azure Policy: If you're using Azure Policy for governance, integrating with Azure RBAC makes policy enforcement more consistent.
- You're new to Kubernetes: Azure RBAC may be easier to learn for teams familiar with Azure but new to Kubernetes.
Enabling Microsoft Entra ID Integration with AKS
Integration with Microsoft Entra ID (formerly Azure AD) benefits both Kubernetes RBAC and Azure RBAC. Let's examine how to set up this integration.
There are two methods for enabling Microsoft Entra ID integration with AKS: the legacy approach and the managed approach. The managed approach is recommended for most scenarios, and we'll focus on it here.
To create a new AKS cluster with managed Microsoft Entra ID integration:
az aks create \
--resource-group myResourceGroup \
--name myAKSCluster \
--enable-aad \
--aad-admin-group-object-ids <admin-group-object-id> \
--generate-ssh-keys
To update an existing AKS cluster to enable managed Microsoft Entra ID integration:
az aks update \
--resource-group myResourceGroup \
--name myAKSCluster \
--enable-aad \
--aad-admin-group-object-ids <admin-group-object-id>
The --aad-admin-group-object-ids parameter specifies a Microsoft Entra ID group with cluster-admin privileges on the cluster. This is necessary to ensure that someone can still administer the cluster after enabling Microsoft Entra ID integration.
Authentication Flow
When Microsoft Entra ID integration is enabled, the authentication flow works as follows:
- A user runs kubectl to access the cluster.
- kubectl contacts the AKS API server and receives a login URL and a device code.
- The user navigates to the login URL, enters the device code, and authenticates with Microsoft Entra ID.
- Microsoft Entra ID issues a token to the user.
- kubectl presents this token to the AKS API server.
- The AKS API server validates the token with Microsoft Entra ID.
- The AKS API server authorizes the request based on the token and the applicable RBAC policies (either Kubernetes RBAC or Azure RBAC).
This flow is similar for both Kubernetes RBAC and Azure RBAC, with the main difference being how the authorization decision is made in step 7.
Obtaining Kubernetes Credentials
To access an AKS cluster with Microsoft Entra ID integration, users need to get the Kubernetes credentials using the az aks get-credentials command:
az aks get-credentials \
--resource-group myResourceGroup \
--name myAKSCluster
When a user runs this command, they are prompted to authenticate with Microsoft Entra ID in their browser. After successful authentication, their Kubernetes configuration file (~/.kube/config) is updated with the appropriate credentials.
For administrator access (bypassing Microsoft Entra ID), you can use the --admin flag:
az aks get-credentials \
--resource-group myResourceGroup \
--name myAKSCluster \
--admin
This is useful for emergency access or for setting up the initial RBAC policies if you're using Kubernetes RBAC but be very careful when you're allowing this capability as if you want to boot users from the clusters, you will need to rotate the credentials which will cause downtime.
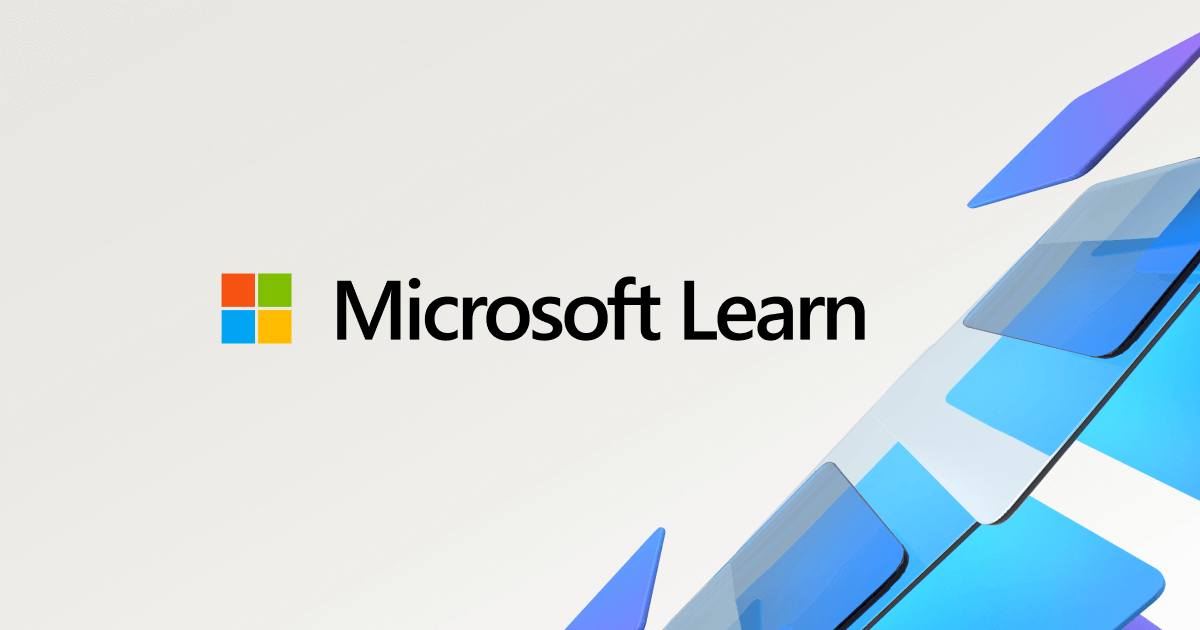
Best Practices for AKS Authorization
Regardless of which authorization model you choose, here are some best practices I've developed over years of working with AKS:
Follow the Principle of Least Privilege
Always grant users and applications the minimum permissions necessary to perform their functions. This reduces the potential impact of compromised credentials.
For example, if a developer only needs to view logs and debug applications in a specific namespace, don't give them write access to that namespace or any access to other namespaces.
Use Groups Instead of Individual Assignments
Assign permissions to groups rather than individual users. This makes it easier to manage access as people join, leave, or change roles within your organization.
In Microsoft Entra ID, create groups that reflect your organizational structure and assign roles to them. Then, manage user permissions by adding users to or removing them from the appropriate groups.
Regularly Audit and Review Permissions
Periodically review who has access to your AKS clusters and what they can do. Remove any unnecessary permissions and ensure that access is still appropriate for each user and application.
For Azure RBAC, you can use Azure Activity Logs to monitor role assignments. For Kubernetes RBAC, consider tools like kubectl auth can-i and third-party auditing solutions.
Implement Proper Namespace Isolation
Use namespaces to isolate different teams, applications, or environments from each other. Each namespace should have its own set of RBAC policies.
For example, create separate namespaces for development, staging, and production environments and ensure that developers can't modify resources in the production namespace.
Secure Service Accounts
Service accounts are used by applications running within the cluster. Apply the principle of least privilege to service accounts as well, giving each application only the permissions it needs.
Consider using Kubernetes' built-in service account token volume projection feature to create time-bound tokens with automatic rotation.
Document Your Authorization Strategy
Document your authorization strategy, including which roles exist, what permissions they grant, and who should have which roles. This documentation should be reviewed and updated regularly.
Include procedures for requesting additional permissions or new roles, as well as the approval process for such requests.
Implement a Break-Glass Procedure
Have a documented procedure for emergency access to your clusters. This might involve a highly privileged service account or user identity that is normally not used but can be activated in case of an emergency.
Ensure that these emergency credentials are heavily audited and that the credentials are secured appropriately.
Advanced Security Considerations with RBAC
After implementing your basic authorization model, it's time to examine some of the security pitfalls that can catch even experienced engineers off guard. I've encountered these issues repeatedly when auditing client environments, and they often go undetected until it's too late.
Common RBAC Security Pitfalls
One of the most critical aspects to understand about Kubernetes RBAC is that it only controls access to the Kubernetes API resources, not what happens directly on the cluster nodes. This distinction leads to one of the most common security vulnerabilities I've seen in production environments.
Horizontal Privilege Escalation via Cluster Nodes
Let's examine a scenario I encountered at a client some time ago. They had meticulously configured namespace isolation using Kubernetes RBAC, believing this provided strict multi-tenancy. However, their security model had a critical flaw.
Consider this situation: You have a cluster with multiple teams, each working in its own namespace. You've configured roles that allow developers to create pods only in their own namespace. This seems secure at first glance, but here's the problem - if those developers can create privileged pods, they can gain access to the underlying node, and from there, potentially access resources in other namespaces.
apiVersion: v1
kind: Pod
metadata:
name: privileged-pod
namespace: team-a
spec:
containers:
- name: privileged-container
image: alpine
command: ["/bin/sh", "-c", "sleep 1000000"]
securityContext:
privileged: true
A user with permissions to create this pod in their namespace could then execute commands like:
kubectl exec -it privileged-pod – /bin/sh
# Now with privileged access, they could access other pods on the same node
# For instance, mounting the host filesystem
mount /dev/sda1 /mnt
# Then browsing to other containers' volumes
ls -la /mnt/var/lib/kubelet/pods/
The solution? Always implement Pod Security Standards (the successor to Pod Security Policies) to restrict what containers can do, regardless of which namespace they're in.
Overly Permissive ClusterRoles
Another common mistake I see is creating overly permissive ClusterRoles to "make things work quickly." This is particularly dangerous because ClusterRoles operate at the cluster level, not just within a namespace.
Let's look at a problematic example I found in a production environment:
apiVersion: rbac.authorization.k8s.io/v1
kind: ClusterRole
metadata:
name: developer-access
rules:
- apiGroups: ["", "apps", "batch", "extensions"]
resources: ["*"]
verbs: ["get", "list", "watch", "create", "update", "patch", "delete"]
This ClusterRole effectively gives users full access to most resources across the entire cluster! Instead, you should scope permissions tightly. Here's an improved version:
apiVersion: rbac.authorization.k8s.io/v1
kind: Role # Note: Not a ClusterRole
metadata:
name: developer-access
namespace: team-a
rules:
- apiGroups: ["", "apps"]
resources: ["deployments", "services", "pods"]
verbs: ["get", "list", "watch", "create", "update"]
- apiGroups: [""]
resources: ["configmaps", "secrets"]
verbs: ["get", "list"]
This more specific role gives developers just what they need, nothing more. Remember, with security, always follow the principle of least privilege.
Service Account Tokens and Secret Management
Another area where I frequently see security issues is with service account tokens. By default, every pod in Kubernetes gets mounted with the default service account token of its namespace. If that service account has been granted excessive permissions, this can lead to unintended access.
Consider this dangerous binding I encountered:
apiVersion: rbac.authorization.k8s.io/v1
kind: ClusterRoleBinding
metadata:
name: default-admin
subjects:
- kind: ServiceAccount
name: default
namespace: default
roleRef:
kind: ClusterRole
name: cluster-admin
apiGroup: rbac.authorization.k8s.io
This gives admin access to the default service account - which means every pod in the default namespace automatically has admin rights to the entire cluster.
The fix is twofold:
- Never bind powerful roles to default service accounts
- Use the automountServiceAccountToken: false setting in pod specs when the pod doesn't need API access
apiVersion: v1
kind: Pod
metadata:
name: my-pod
spec:
automountServiceAccountToken: false
containers:
- name: main-container
image: myapp:1.0
Troubleshooting Authorization Issues in AKS
One of the most frustrating experiences you can have with Kubernetes is troubleshooting authorization issues. Let me walk through some common problems and their solutions.
Role Assignments Not Taking Effect
A common issue with clients is when role assignments don't take effect immediately. This can be particularly confusing for Kubernetes with Azure RBAC.
The Problem: You've assigned a role to a user, but they still get "forbidden" errors when trying to access resources.
The Solution: Azure RBAC role assignments can take up to 5 minutes to propagate. This is because resource providers (like Microsoft Storage) are notified of the update and need to update their local caches with the latest role assignments. If you've just made a role assignment change, wait for about 5 minutes and try again.
Here's a command I use to check if a role assignment is correctly set up:
# For Azure RBAC
az role assignment list --assignee [email protected] --scope /subscriptions/<subscription-id>/resourceGroups/<resource-group>/providers/Microsoft.ContainerService/managedClusters/<cluster-name>
# For Kubernetes RBAC
kubectl auth can-i get pods [email protected] -n some-namespace
Debugging with Impersonation
One of my favorite tricks when troubleshooting RBAC issues is using the impersonation feature in Kubernetes. This allows cluster administrators to simulate actions as if they were another user:
# Check if a specific user can list pods in a namespace
kubectl auth can-i list pods --namespace development --as [email protected]
# Try to execute an actual command as another user
kubectl get pods --namespace development --as [email protected]
This is incredibly useful for verifying your RBAC configurations without needing to log in as different users.
Solving Azure RBAC Condition Issues
When working with Azure RBAC conditions (a more advanced feature), you might encounter the error The given role assignment condition is invalid. This typically happens for two reasons:
- The conditionVersion property is set to "1.0" instead of "2.0"
- Your condition has syntax issues
To fix this, ensure your condition version is set to "2.0" and verify the syntax of your condition. Azure RBAC conditions allow you to apply additional constraints on role assignments, like restricting access to specific resources based on tags or other attributes.
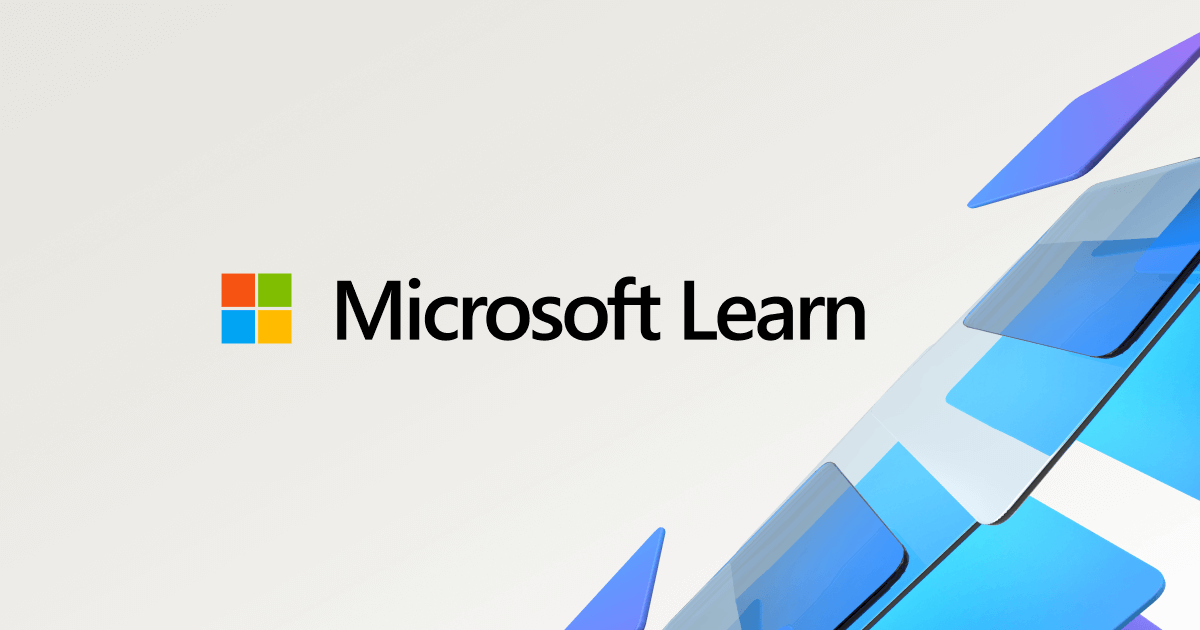
Implementing Enterprise-Grade AKS Authorization Models
Having explored the technical details of both authorization models, let's now look at how to implement them in enterprise scenarios.
Hybrid Approach: Combining Azure RBAC and Kubernetes RBAC
I often take a more hybrid approach when facing complex requirements, that leverages the strengths of both authorization models.
Here's how it works:
- Use Azure RBAC for cluster-level access control:
- Control who can access the cluster and the Kubernetes API
- Manage access to different namespaces at scale
- Leverage existing Azure role assignments and Microsoft Entra ID integration
- Use Kubernetes RBAC for fine-grained permissions within namespaces:
- Create custom roles for specific application needs
- Define granular permissions for service accounts used by applications
- Implement specialized role bindings for edge cases not covered by Azure RBAC
This hybrid approach combines the seamless integration and management of Azure RBAC with the flexibility and power of Kubernetes RBAC.
Implementing Team-Based Isolation
For larger enterprises with multiple development teams, I typically recommend implementing a team-based isolation model with the following structure:
- Namespace per Team:
- Each team gets one or more dedicated namespaces
- Namespace names follow a standard pattern: <business-unit>-<team>-<environment>
- Example: finance-payments-dev, finance-payments-prod
- Resource Quotas for Each Namespace:
- Prevent any single team from consuming all cluster resources
- Encourage efficient resource usage
apiVersion: v1
kind: ResourceQuota
metadata:
name: team-quota
namespace: team-a-dev
spec:
hard:
requests.cpu: "10"
requests.memory: 20Gi
limits.cpu: "20"
limits.memory: 40Gi
pods: "50"
- Network Policies for Isolation:
- Default deny all ingress/egress
- Explicitly allow only required traffic
apiVersion: networking.k8s.io/v1
kind: NetworkPolicy
metadata:
name: default-deny
namespace: team-a-dev
spec:
podSelector: {}
policyTypes:
- Ingress
- Egress
- Role-Based Access Control Hierarchy:
- Team Admins: Full access to team namespaces
- Team Developers: Read/write access to non-sensitive resources
- Team Viewers: Read-only access
- Platform Admins: Cluster-wide access
This structured approach scales well for enterprises with dozens or even hundreds of teams.
Audit Logging and Monitoring for AKS Authorization
Implementing robust authorization is only half the battle - you also need to monitor and audit who's doing what in your cluster. Let me share some best practices I've implemented for AKS audit logging.
Setting Up Comprehensive Audit Logs
AKS provides robust audit logging capabilities that you should enable to maintain visibility into your cluster activities:
# Enable Azure Monitor for Containers with audit logs
az aks update \
--resource-group myResourceGroup \
--name myAKSCluster \
--enable-azure-monitor
Specifically, enable the following audit logs:
- kube-audit: Captures all API requests to the Kubernetes API server
- kube-controller-manager: Logs actions performed by the controller manager
- kube-apiserver: Records API server activities
These logs should be exported to Azure Monitor Log Analytics for historical analysis and retention:
# Configure diagnostic settings to send logs to Log Analytics
az monitor diagnostic-settings create \
--resource $(az aks show -g myResourceGroup -n myAKSCluster --query id -o tsv) \
--name "aks-diagnostics" \
--workspace $(az monitor log-analytics workspace show -g myResourceGroup -n myWorkspace --query id -o tsv) \
--logs '[{"category":"kube-audit","enabled":true},{"category":"kube-apiserver","enabled":true},{"category":"kube-controller-manager","enabled":true},{"category":"kube-audit-admin","enabled":true},{"category":"kube-scheduler","enabled":true},{"category":"cluster-autoscaler","enabled":true},{"category":"cloud-controller-manager","enabled":true}]'
Setting Up Alerts for Suspicious Activities
Once you have logging in place, set up alerts for suspicious activities such as:
- Multiple failed authentication attempts
- Changes to RBAC configurations
- Creation of privileged containers
- Access to sensitive secrets
Here's a sample KQL query to detect changes to RBAC configurations:
AzureDiagnostics
| where Category == "kube-audit"
| where log_s contains "rbac.authorization.k8s.io"
| where log_s contains "\"verb\":\"create\"" or log_s contains "\"verb\":\"update\"" or log_s contains "\"verb\":\"delete\""
| project TimeGenerated, log_s
Setting up automated alerts based on these queries can help you respond quickly to potential security issues.
AKS Authorization in Hybrid and Multi-Cloud Environments
Many enterprises today operate in hybrid or multi-cloud environments. Let's explore how to extend AKS authorization models to these scenarios.
Azure Arc-Enabled Kubernetes
Azure Arc allows you to extend Azure's management capabilities to any Kubernetes cluster, including those running outside of Azure. You can use Azure RBAC for Kubernetes authorization on Azure Arc-enabled clusters, giving you a consistent authorization model across your entire Kubernetes estate.
To enable Azure RBAC on an Azure Arc-enabled Kubernetes cluster:
# First, connect your Kubernetes cluster to Azure Arc
az connectedk8s connect --name myCluster --resource-group myResourceGroup
# Then, enable Azure RBAC
az connectedk8s enable-features --name myCluster --resource-group myResourceGroup --features azure-rbac
# Get the cluster MSI identity
clusterObjectId=$(az connectedk8s show -g myResourceGroup -n myCluster --query identity.principalId -o tsv)
# Assign the necessary role to the cluster MSI
az role assignment create --role "Connected Cluster Managed Identity CheckAccess Reader" --assignee-object-id $clusterObjectId --assignee-principal-type ServicePrincipal
Once enabled, you can assign Azure roles to users and groups just as you would for an AKS cluster, providing a consistent experience across your entire Kubernetes fleet.
Considerations for Multi-Cloud Environments
When operating across multiple cloud providers, consistency becomes a major challenge. Here are some strategies I've used successfully:
- Use Kubernetes RBAC for Cross-Cloud Consistency:
- Kubernetes RBAC works the same way across all Kubernetes distributions
- Create identical RBAC configurations across all clusters
- Use a GitOps approach to ensure configurations remain in sync
- Federate Identity Across Clouds:
- Use a single identity provider across all environments
- Consider federated identity solutions that work with multiple clouds
- Implement consistent naming conventions for groups and roles
- Standardize on Tools and Processes:
- Use the same deployment and management tools across all clusters
- Create consistent processes for requesting and granting access
- Implement the same monitoring and alerting solutions
Conclusion
Authorization isn't a "set it and forget it" thing. It's an ongoing process that needs regular attention. I've seen too many organizations implement a solid authorization model initially, only to let it degrade over time as they add exceptions and one-off permissions to "just make it work."
Instead, when you need to make changes, take the time to understand the underlying need and adjust your model appropriately. Your future self will thank you when you don't have to untangle a mess of random permissions a year from now.
So, to summarize:
- Start with the principle of least privilege - grant only the permissions each user or service account actually needs.
- Leverage Microsoft Entra ID integration for seamless identity management across your Azure estate.
- Choose the right authorization model based on your specific requirements and existing investments in Azure.
- Implement robust monitoring and auditing to detect and respond to potential security issues.
- Regularly review and refine your authorization model as your applications and organization evolve.
That being said, I hope this helps. Remember, there's no perfect solution - just the one that works best for your specific needs.
As always, have a good one!